There are times when you want to add html tag attributes in a React app.
We normally add attributes based on some logic, for example if some state value is true set the value of the attribute as something.
Example
<div className={ this.state.isRisk ? "danger" : "normal">...</div>
This will be either rendered as
<div class="danger">...</div>
ordiv class="normal">...</div>
based on the state value isRisk
In the above case there is a value for the attribute, that is class="danger"
. Sometimes you don’t want to have any value for the attribute. I was using a third party library which checks only if the attribute is present, it does not care about the attribute values.
So I tried something like this.
<div { this.state.isPrint ? data-is-print : null } >...</div>
I am using vscode editor and it will immediately throw the below error.
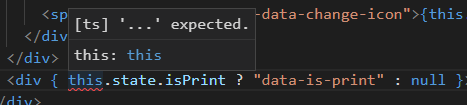
vscode error when trying to add attribute with empty values
I need the output like
<div data-is-print>...</div>
This is how to do that.
Have a variable like this.
let dataIsPrint = this.state.isPrint ? { "data-is-print" : "" } : {};
In the render()
use like this.
<div { ...dataIsPrint } > Some text </div>
The rendered code will look like this.
<div data-is-print> Some text </div>
The same method can be used to add checked
and disabled
attributes in form inputs.
Hope this helps… 🍹
One Comment
Jesús
You can also just make it equal to an empty string:
Some text
Worked for me.
Thanks for helping me figure it out, though.